CSC 112 Past Question and Answers
CSC 112 Past Question and Answers
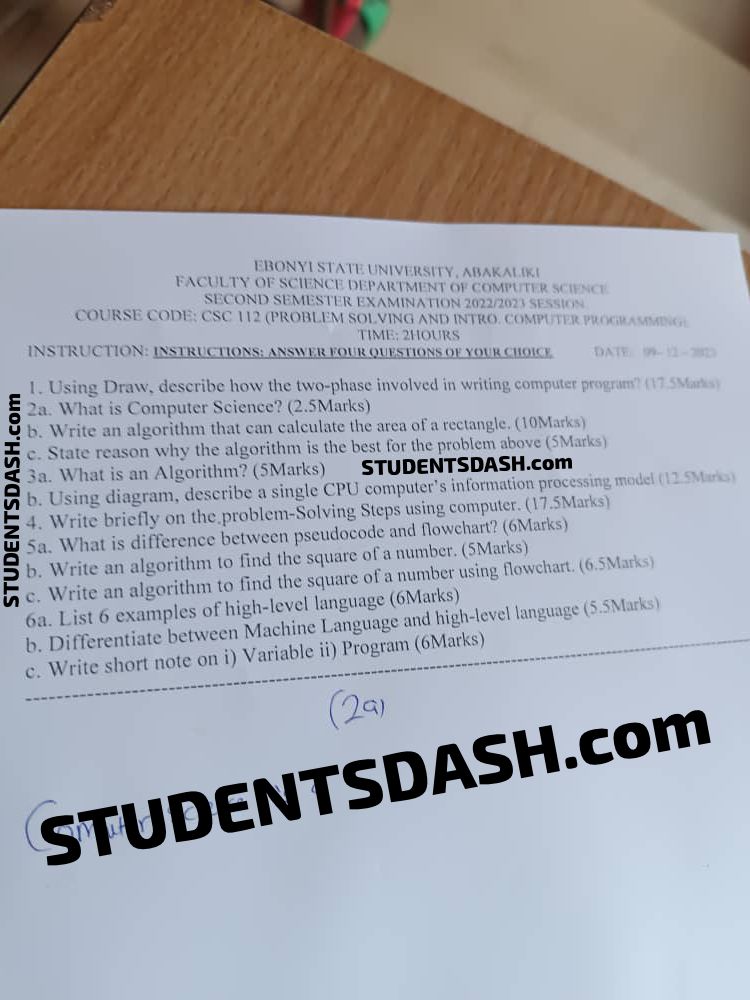

CSC 112 SOLUTION
Question 1
(a) Problem Solving and its Relation to Computer Science: Problem solving is a cognitive process that involves discovering, analyzing, and solving problems. It is a skill that individuals use to address challenges and achieve goals. In the context of computer science, problem solving is fundamental to the field. Computer scientists encounter various complex problems, ranging from designing efficient algorithms to troubleshooting software bugs.
Problem solving in computer science often involves breaking down a larger problem into smaller, more manageable components. It requires analytical thinking, creativity, and the ability to develop systematic approaches to find solutions. Computer scientists use problem-solving techniques to design algorithms, develop software, optimize systems, and address various computational challenges.
(b) Stages in Problem Solving:
(i) Define the Problem:
- Understanding the Problem: The first stage is to gain a clear understanding of the problem. This involves gathering relevant information, identifying constraints, and defining the scope of the problem.
- Analyzing the Problem: Break down the problem into smaller parts to identify its components. Analyzing helps in understanding the relationships between different elements and the overall structure of the problem.
- Identifying Constraints and Requirements: Determine any limitations or constraints that must be considered when devising a solution. Identify the requirements that the solution must meet.
(ii) Generate Alternative Solutions:
- Brainstorming: Encourage the generation of a wide range of ideas without evaluating them initially. This fosters creativity and ensures that various perspectives are considered.
- Research and Gather Information: Look for existing solutions or approaches that might be relevant to the problem. This may involve studying literature, consulting experts, or reviewing similar problems and their solutions.
- Evaluate and Select Solutions: Assess the feasibility, efficiency, and effectiveness of each alternative solution. Choose the solution that best meets the defined criteria and requirements.
Question 2
(a) Three Basic Levels of Programming Languages:
- Machine Language (Low-Level Language):
- Explanation: Machine language is the lowest-level programming language, consisting of binary code that directly corresponds to the computer’s hardware instructions. It is machine-specific and challenging for humans to read and write.
- Characteristics: It is hardware-dependent, difficult to program, and directly executed by the computer’s central processing unit (CPU).
- Example: Binary code representing instructions such as 01011001.
- Assembly Language (Low-Level Language):
- Explanation: Assembly language is a symbolic representation of machine language using mnemonics and symbols. It is specific to a particular computer architecture and provides a more human-readable format than machine language.
- Characteristics: It is still closely tied to the computer’s architecture but is more readable than machine language. It requires an assembler to convert it into machine code.
- Example: MOV A, B (represents a move operation in assembly language).
- High-Level Language:
- Explanation: High-level languages are designed to be more user-friendly and independent of the computer’s hardware. They use English-like syntax and are closer to human language. High-level languages need a compiler or interpreter to convert code into machine language.
- Characteristics: Easier to learn and write, more abstract, and portable across different platforms.
- Examples: Python, Java, C++, and Ruby.
(b) Factors to Consider in Programming:
(i) Anxiety:
- Explanation: Anxiety can significantly impact a programmer’s performance. It may arise from tight deadlines, fear of making mistakes, or the complexity of the task at hand.
- Considerations:
- Break Down Tasks: Divide the programming tasks into smaller, more manageable components to reduce anxiety.
- Seek Support: Encourage collaboration and seek support from colleagues or online communities.
- Time Management: Effectively manage time to alleviate the pressure associated with tight deadlines.
- Learning Mindset: Embrace a growth mindset and view challenges as opportunities for learning rather than sources of anxiety.
(ii) Time Management:
- Explanation: Time management is crucial in programming to meet project deadlines and maintain productivity.
- Considerations:
- Prioritization: Identify and prioritize tasks based on their importance and urgency.
- Break Down Tasks: Divide complex tasks into smaller, more manageable subtasks to facilitate efficient progress.
- Set Realistic Goals: Establish achievable goals and deadlines to avoid feeling overwhelmed.
- Use Tools: Utilize project management and version control tools to streamline workflows and collaboration.
- Regular Breaks: Schedule regular breaks to prevent burnout and maintain focus.
Question 3
(a) Programming Languages: Object-oriented and Procedural
(i) Object-oriented Languages:
- Explanation: Object-oriented programming (OOP) is a programming paradigm that organizes code into objects, each encapsulating data and the functions that operate on that data. Objects interact through well-defined interfaces, promoting modularity and code reusability.
- Characteristics:
- Encapsulation: Bundling of data and methods that operate on the data within a single unit (object).
- Inheritance: Allows a class (a blueprint for objects) to inherit properties and behaviors from another class, promoting code reuse.
- Polymorphism: The ability of objects of different classes to respond to the same message or method call in a way that is appropriate for their class.
- Abstraction: Hiding the complexity of the internal workings of an object and exposing only what is necessary.
- Examples: Java, C++, Python, and Ruby.
(ii) Procedural Programming Languages:
- Explanation: Procedural programming is a paradigm where the program is structured around procedures or routines that are invoked to perform tasks. It emphasizes procedures (functions or routines) that manipulate data, and the flow of control is directed by procedure calls.
- Characteristics:
- Top-down Design: Decomposing a program into smaller, manageable procedures.
- Data Focus: Emphasis on data structures and the procedures that operate on them.
- Sequential Execution: Execution proceeds in a linear manner, following the order of statements.
- Modularity: Breaking down a program into smaller, independent modules.
- Examples: C, Fortran, and Pascal.
(b) Program Control Structure:
- Explanation: Program control structure refers to the way in which the flow of control is organized in a program. It determines the order in which statements are executed based on specified conditions or loops.
- Types of Program Control Structures:
- Sequential Structure: Statements are executed in a sequential, linear order.
- Selection Structure (Conditional Structure): Decisions are made based on conditions, and different paths are followed accordingly (e.g., if statements).
- Repetition Structure (Loop Structure): Execution of statements is repeated as long as a specified condition is true (e.g., for loops, while loops).
- Importance:
- Efficient control structures enhance code readability and maintainability.
- They provide mechanisms for handling different scenarios based on conditions.
- They allow the creation of repetitive structures, reducing redundancy in code.
- Example (Selection Structure in Python):
age = 25 if age >= 18: print("You are eligible to vote.") else: print("You are not eligible to vote.")
In this example, the program flow diverges based on the condition (age >= 18), illustrating a selection structure.
SECTION B
Question 1
Method 1: Pseudocode
1. Start
2. Check if the lamp is plugged in.
a. If not, plug in the lamp.
3. Check if the bulb is burnt out.
a. If yes, replace the bulb.
4. Inspect the power cord for any damage.
a. If damaged, repair or replace the power cord.
5. Check the switch for functionality.
a. If the switch is faulty, repair or replace it.
6. If all components are in working order, turn on the lamp.
7. End
Method 2: Flowchart
graph TD
A[Start] --> B{Is the lamp plugged in?}
B -- No --> C[Plug in the lamp]
B -- Yes --> D{Is the bulb burnt out?}
D -- Yes --> E[Replace the bulb]
D -- No --> F{Is the power cord damaged?}
F -- Yes --> G[Repair or replace power cord]
F -- No --> H{Is the switch faulty?}
H -- Yes --> I[Repair or replace the switch]
H -- No --> J[Turn on the lamp]
J --> K[End]
Question 2
- Define the Problem:
- Clearly articulate the problem in detail.
- Understand the requirements and constraints associated with the problem.
- Gather relevant information and data related to the issue.
- Analyze the Problem:
- Break down the problem into smaller, more manageable components.
- Identify the relationships between different elements of the problem.
- Understand the context and potential causes of the problem.
- Specify Requirements:
- Define the criteria that a solution must meet.
- Identify any constraints, limitations, or specific needs.
- Develop a Solution:
- Design a solution based on the analysis.
- Choose appropriate algorithms, data structures, and methodologies.
- Consider modularity, code reusability, and maintainability.
- Implement the Solution:
- Write the code or develop the software based on the designed solution.
- Pay attention to coding standards and best practices.
- Debug and test the solution iteratively.
- Test the Solution:
- Verify that the implemented solution meets the specified requirements.
- Conduct various tests to ensure the correctness and reliability of the solution.
- Handle edge cases and exceptions.
- Evaluate and Refine:
- Assess the effectiveness of the solution in addressing the problem.
- Gather feedback from users or stakeholders.
- Refine the solution based on feedback and any additional insights.
- Document the Solution:
- Create documentation that explains the solution, its implementation, and any relevant considerations.
- Include comments in the code for future reference.
- Document any potential issues or areas for improvement.
- Deploy the Solution:
- Integrate the solution into the relevant environment or system.
- Ensure proper configuration and compatibility.
- Monitor the solution’s performance after deployment.
- Maintain and Update:
- Address any issues or bugs that may arise post-deployment.
- Keep the solution up-to-date with changes in requirements or technology.
- Continuously improve the solution based on user feedback and evolving needs.
- Reflect and Learn:
- Conduct a post-implementation review to reflect on the problem-solving process.
- Identify lessons learned and areas for improvement.
- Apply insights from the problem-solving experience to future challenges.
Question 3
Share with your friends nd course mates.